COCO 형태의 train, val, test JSON파일 안에는 여러 클래스가 있다. 이 중 내가 특정 경우에 일부 클래스만 학습하고자 할 때 해당 Json파일을 수정할 수 있도록 해주는 오픈소스가 있어 공유한다. https://github.com/immersive-limit/coco-manager GitHub - immersive-limit/coco-manager: Scripts to manage COCO datasets Scripts to manage COCO datasets. Contribute to immersive-limit/coco-manager development by creating an account on GitHub. github.com 간단하게 원하는 input file, outp..
사소한 Tip . 오류 해결법/python
dictionary, list, np arr같은 python 객체를 파일로 binary파일로 저장하여 빠른 속도 처리가 가능하도록 함. pkl file reading # Data Loading import pickle import os data_dir = './dataset/pkl_dir/' file_lst = os.listdir(data_dir) # directory안에 있는 모든 pkl파일을 다 로딩해서 그 중 하나만 load with open(os.path.join(data_dir, file_lst[0]), 'rb') as fr: # 여기선 0-index(첫번째)파일을 로드 data = pickle.load(fr) print(data) print(data.keys()) pkl file writing..
pdf 파일에서 페이지를 삭제하거나 PDF를 합병하고 싶을 때 다음 코드를 사용해서 돌리면 pdf파일을 내 맘대로 수정할 수 있다. 아래 코드는 한 부당 7페이지로 구성된 40부짜리 문서 중 앞에 있는 3부만 잘라내어 저장하는 스크립트다. import PyPDF2 if __name__ == "__main__": # target_path : pdf 문서가 있는 경로 target_path = "./문서_40부.pdf" pdf = PyPDF2.PdfReader(target_path) output = PyPDF2.PdfWriter() pageNum = len(pdf.pages) oneDoc = 7 # 한 부당 페이지 개수 for i in range(0, 3*oneDoc):#처음에 있는 3부만 따로 저장 page..
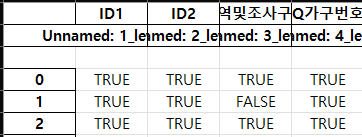
Multiindex를 갖고 있는 데이터 프레임을 그대로 excel로 떨어트리는 함수를 사용할 경우에 제목 행 바로 아래에 빈 행이 하나 생기게 된다. 이를 해결하기 위한 함수는 아래와 같다. 간단히, 제목만 떼서 저장하고, 이어서 내용에 해당되는 부분만 떼서 2번에 걸쳐 저장하는 함수라 보면 된다. def save_double_column_df(df, file_name, startrow = 0, **kwargs): '''Function to save doublecolumn DataFrame, to xlwriter''' # https://stackoverflow.com/questions/52497554/blank-line-below-headers-created-when-using-multiindex-and..
import os from PIL import Image directory = "./images" image_files = natsorted([filename for filename in os.listdir(directory) if filename.endswith('.jpg')]) images = [Image.open(directory + '/' + f) for f in image_files] images[0].save("out.pdf", "PDF", resolution=100.0, save_all=True, append_images=images[1:]) # 1부터 저장해야지 안그러면 똑같은 페이지 하나 더 들어감
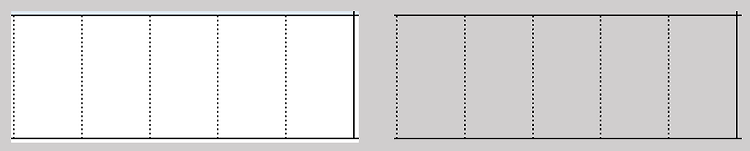
remain only text or black thing. remove backgrounds 배경은 삭제하고, 검은 영역만 남기기 import cv2 import numpy as np from PIL import Image, ImageFilter, ImageOps import glob list = glob.glob("./*") list.remove("./transparent.py") # want to make transparent image. # turn background as transparent. # only remain black text. for item in list: img = Image.open(item).convert("RGBA") nm = item.split("/")[-1] datas ..
open() got an unexpected keyword argument 'encoding' Json파일을 open해서 load하는 과정에서 encoding에러가 나길래, open함수 인자로 encoding 옵션을 넣어주었더니 상단과 같은 에러가 발생했다. 왜 없어진건진 모르겠지만 기존 open함수에 인자로 넣어주던 인코딩 옵션이 python 3.9로 업그레이드 되면서 사라졌다고 한다. python을 3.8로 다운그레이드 하거나, 아니면 아래 방법으로 변경할 수는 있다고 한다. def loads(txt): txt = txt.encode("utf-8") value = json.loads(txt) return value https://stackoverflow.com/questions/67723694/pyt..
Object Detection같은 수십개의 클래스에 대하여, 클래스 별 color를 다 다르게 맵핑하여 시각화 할 경우에는 다음 코드로 팔레트를 정의할 수 있다. CLASSES = [ A, B, C, D, ..., Z ] colors = np.random.uniform(0, 255, size=(len(CLASSES), 3))
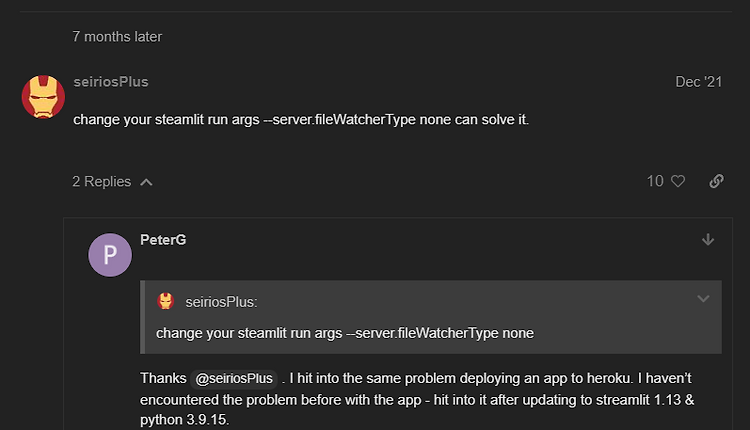
streamlit 설치 후, 테스팅해보는데 다음과 같은 에러 발생. # 기존 명령어 $ streamlit hello # 새로운 명령어 $ streamlit hello --server.fileWatcherType none 기존 명령어에 아래 옵션을 붙여주면 해결. --server.fileWatcherType none https://discuss.streamlit.io/t/oserror-errno-24-inotify-instance-limit-reached/5506/5 OSError: [Errno 24] inotify instance limit reached Cheerful!! I find a solution after nearly one year. Other solution just change max_u..
https://stackoverflow.com/questions/6482889/get-random-sample-from-list-while-maintaining-ordering-of-items Get random sample from list while maintaining ordering of items? I have a sorted list, let say: (its not really just numbers, its a list of objects that are sorted with a complicated time consuming algorithm) mylist = [ 1 , 2 , 3 , 4 , 5 , 6 , 7 , 8 ,9 , 10 ] ... stackoverflow.com sample_s..
https://stackoverflow.com/questions/75409654/python-valueerror-the-truth-value-of-an-array-with-more-than-one-element-is-am Python, ValueError: The truth value of an array with more than one element is ambiguous. Use a.any() or a.all() My question is related to the answer to my previous question. the difference between the code in the previous solutions and the current code on this question is a..
https://aihints.com/how-to-draw-bounding-box-in-opencv-python/ How to Draw Bounding Box in OpenCV Python - AiHints You can draw a bounding box in OpenCV Python by following the given steps. Import the OpenCV library. Now read the image from the location. aihints.com 이미지 내 특정 그림을 포함하는 bbox만을 남겨두고, 나머지는 crop해버리는 코드 def make_inner_crop(): # target_lst is list of cropped image, # we're going to remo..
단순 기록용 포스팅입니다. 원 출처는 아래를 참고 부탁드립니다. pip install googletrans==4.0.0-rc1 def korean_to_eng(text): translator = Translator() name2eng = translator.translate(text, dest='en').text return name2eng - 도움 주신 출처 : https://coding-kindergarten.tistory.com/98 [Python/Googletrans] 파이썬으로 구글 번역 API 사용하는 법 안녕하세요, 왕초보 코린이를 위한 코딩유치원에 오신 것을 환영합니다. 오늘은 영어를 해석할 때 자주 도움받는 구글 번역(Google Translate)을 파이썬에서 사용하는 방법에 대해 알아..
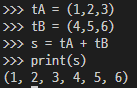
기본적으로 tuple 내 합은 concat을 의미한다. 만약 길이가 동일한 tuple끼리 더하여, 요소 합을 만들고 싶으면 아래 처럼 진행하면 된다. sum = tuple(sum(elem) for elem in zip(tA, tB)) 도움 주신 사이트 : 출처 [tuple] 튜플 요소끼리 더하기, 튜플요소에 상수 곱하기 파이썬에는 array와 유사해 보이는 tuple 이라는 개념이 있다. () 를 사용하여 둘러싸면 tuple이 되는데 사... blog.naver.com
reads the file list.txt in which the file names are listed and deletes the rest leaving only the files that exist in it import os import glob from tqdm import tqdm import pdb # Read the list of filenames from list.txt for step in ["train","val", "test"]: print("=="+step+"==") with open(step+".txt", 'r') as f: filenames = f.read().splitlines() filenames = [f_n.split("/")[-1].rstrip("\n") for f_n ..